Common JavaScript Errors and How to Debug Them
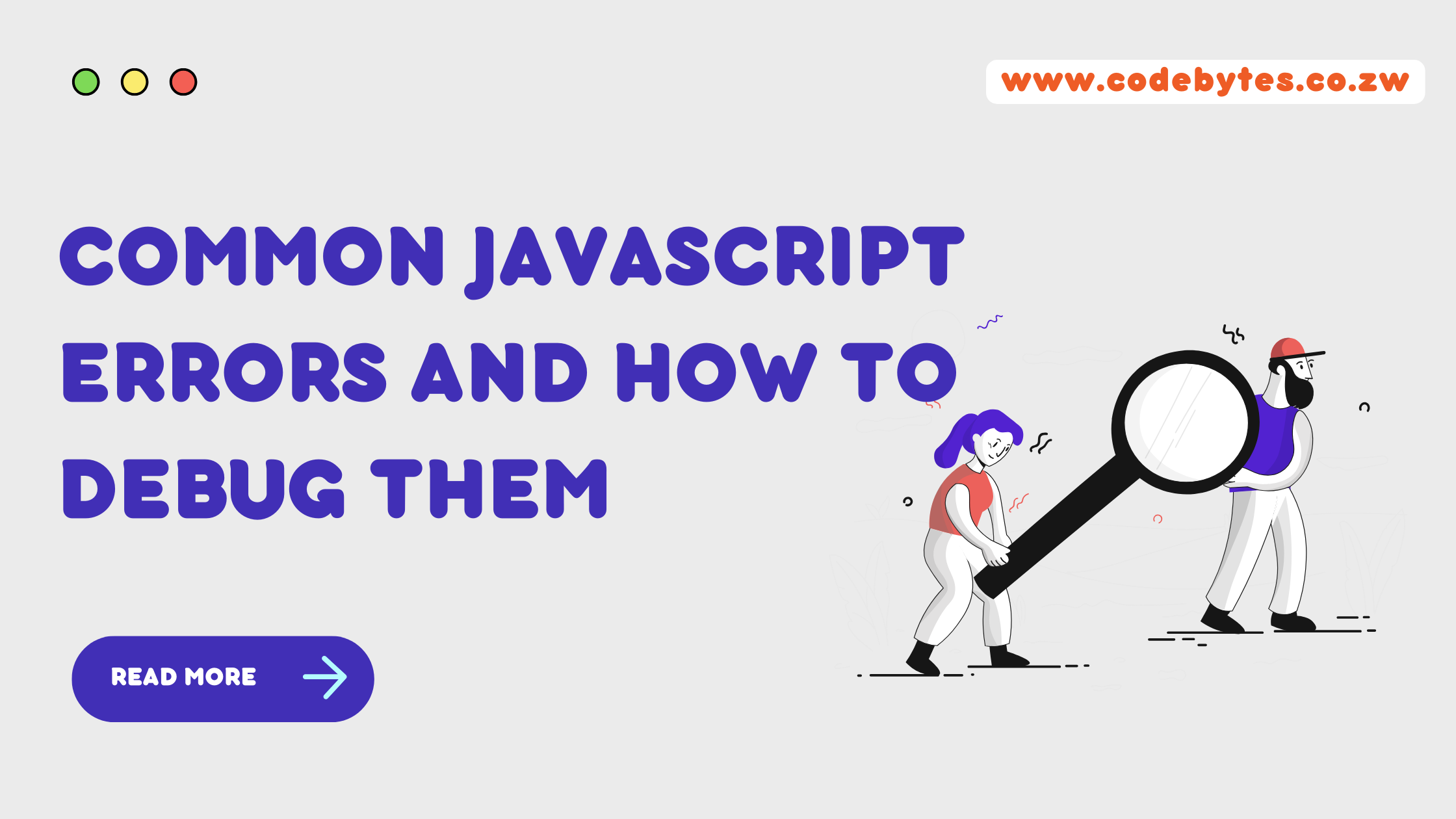
JavaScript is a popular programming language used for web development, but it can sometimes be frustrating to work with. One of the most common sources of frustration is dealing with errors that pop up when running your code. Identifying and fixing these errors can be difficult if you don't know what to look for. They can range from simple syntax errors to more complex issues.
This blog post will cover some of the most common JavaScript errors and how to debug them. We'll also introduce you to tools and techniques that can help you find and fix errors more efficiently. We'll start with the basics and work our way up to more advanced debugging techniques, so whether you're a beginner or an experienced developer, you'll find something helpful in this post.
Common JavaScript Errors
Before we get into debugging, it's helpful to know what types of errors you might encounter when working with JavaScript. Here are some of the most common errors you might see:
Syntax errors
These are mistakes in the structure of your code, such as missing brackets or semicolons. They're often easy to spot, as they'll cause the code to fail to run or produce unexpected results. If you use an good IDE it will probably pick up syntax error as you write your code. Here's an example of a syntax error:
const x = 'hello;
In this code snippet, the string is supposed to be closed with a quotation mark, but it's missing. As a result, the code will produce a syntax error.
ReferenceError
A ReferenceError occurs when you try to access a variable or property that is undefined
. This can happen when you forget to declare a variable, or when you try to access an object property that doesn't exist. Here's an example of a ReferenceError:
Uncaught ReferenceError: foo is not defined
This error occurs if you try to access the foo variable, which has not been defined. To avoid ReferenceErrors, you can make sure to declare all of your variables and check for the existence of object properties before trying to access them.
TypeError
A TypeError occurs when you try to perform an operation on a value that is not of the expected type. For example, you might see a TypeError when you try to call a function on a value that of the wrong type (calling an array method on a integer value), or when you try to access a property of an object that is null
. Here's an example of a TypeError:
TypeError: Cannot read properties of null (reading 'length')
This error occurs if you try to access the length
property of an object that is null
. In this case, the object is null
, so it doesn't have a length
property and the operation fails.
To avoid TypeErrors, you can use type checking to ensure that the values you are working with are of the expected type. There are several ways to do this in JavaScript, including the typeof
operator and the instanceof
operator. Here's an example of how to use these operators:
// Use the typeof operator to check the type of a value.
if (typeof value === "string") {
// The value is a string.
// You can perform string operations here.
} else {
// The value is not a string.
// You can display an error message or handle the value differently.
}
// Use the instanceof operator to check the type of an object.
if (value instanceof Array) {
// The value is an array.
// You can perform array operations here.
} else {
// The value is not an array.
// You can display an error message or handle the value differently.
}
By using type checking, you can ensure that you are working with the correct data types and avoid TypeErrors.
RangeError
A RangeError is thrown when you pass a value to a function that's outside the valid range of that function. For example, a function might only take in positive numbers, if you pass a negative number that function will likely throw a range error. In javascript a range error can be thrown when we try and create a new array of fixed length outside of the range 0 and 232 - 1. See code sample below
const a = new Array(-1);
// Uncaught RangeError: Invalid array length
const b = new Array(30000000000);
// Uncaught RangeError: Invalid array length
A
above we try an create two array a and b, one of length -1 and the other of length 30000000000. Both are outside the given range and both will throw a RangeError. To avoid this make sure you read documentation of function to ensure you call them with proper arguments.
JSON Syntax Errors
JSON (JavaScript Object Notation) is a popular data interchange format that's often used to transmit data between a server and a client. If you try to parse JSON that's not well-formed, you might see a syntax error.
Here's an example of a JSON syntax error:
Uncaught SyntaxError: Unexpected token o in JSON at position 1
This error occurs when the JSON being parsed is not valid. This can be caused by a missing or extra comma, a missing or extra quotation mark, or a missing or extra curly brace.
To fix this error, you'll need to check the JSON and make sure it's well-formed. You can use a tool such as JSONLint to help you identify and fix the problem.
Network Errors
If you try to make an HTTP request and the network is down or the server is unreachable, you might see a network error. Here's an example of a network error:
Uncaught NetworkError: Failed to execute 'send' on 'XMLHttpRequest': Failed to load 'http://example.com/'
This error occurs when the browser is unable to make a connection to the server. This can be caused by a variety of factors, including a poor internet connection, a problem with the server, or a firewall blocking the request.
To check for a network connection and handle network errors, you can use the navigator.onLine
property and the window.addEventListener
method in JavaScript. Here's an example of how to do this:
if (navigator.onLine) {
// The browser has a working internet connection.
// You can make an HTTP request here.
} else {
// The browser does not have a working internet connection.
// You can display an error message or try again later.
window.addEventListener("online", function() {
// The browser has regained a working internet connection.
// You can make an HTTP request here.
});
}
This code will check for a working internet connection and, if one is not found, it will register an event listener that will be triggered when the connection is restored.
Debugging Tools and Techniques
Now that you know what types of errors you might encounter let's look at some tools and techniques you can use to find and fix them. The first and most essential tool is the browser's developer console.
To access the developer console in most browsers, you can use the F12
key or right-click on the page and select "Inspect." This will open up a panel with various tools, including a console where you can see messages and errors generated by the code.
The console is a powerful tool for debugging because it can show you exactly where the error is occurring and give you some information about what caused it. For example, if you see a syntax error, the console will highlight the problematic line of code and tell you what's wrong with it. You can then go in and fix the error by correcting the syntax.
In addition to the console, there are other tools you can use to debug JavaScript errors. For example, you can use a debugger to pause the code at a certain point and step through it line by line. This can help to track down a bug that's difficult to spot. When using a debugger, you'll need to set a breakpoint where you want the code to pause. You can do this by clicking on the line number in the developer console or by using the debugger
keyword in your code.
Once you've set a breakpoint, you can use the debugger's controls to step through the code and see what's happening at each step. You can also use the console to inspect the values of variables and see how they change as the code is executed. This can help you understand what's going wrong and identify the root cause of the error.
Advanced Debugging Techniques
As you become more comfortable with debugging, you might want to try some more advanced techniques. Here are a few to keep in mind:
- Logging: If you're having trouble figuring out what's going wrong, one of the simplest things you can do is add some
console.log()
statements to your code. This will allow you to see what's happening at different points in the code and help you identify where the problem occurs. - Assertions: An assertion is a statement that checks if something is true or not. If the assertion is false, it will throw an error. You can use assertions to validate your code and ensure it's doing what you expect. For example, you might use an assertion to check if a variable is a correct type or if a function is returning the expected result.
- Unit tests: Unit tests are a way of testing individual pieces of code to make sure they're working correctly. They allow you to run a series of tests against your code and see if it's producing the expected results. This can be especially helpful if you're working on a larger project and want to ensure everything is working as it should.
- Debugging libraries: Several libraries and tools are available to help you debug your code. Some popular options include
debug
,node-inspector
, andjest
. These libraries can provide additional features and functionality beyond what's available in the browser's developer console.
Conclusion
Debugging JavaScript errors can be challenging, but it is easier with the right tools and techniques. By familiarizing yourself with common errors and using the tools and techniques outlined in this post, you'll be well on your way to debugging your JavaScript code like a pro.
And if you're looking to improve your debugging skills even further, there are plenty of resources available online to help you. Here are our favourites:
- Stack Overflow: Stack Overflow is a popular Q&A platform where developers can ask and answer questions about programming. It's a great resource for finding solutions to common JavaScript errors and learning from the experiences of other developers.
- Mozilla Developer Network (MDN) JavaScript Reference: MDN is a comprehensive resource for web developers, and their JavaScript reference is a thorough guide to the language. It includes detailed information on every aspect of JavaScript, including syntax, operators, functions, and objects.
- JSHint: JSHint is a static code analysis tool that helps you find and fix errors in your JavaScript code. It checks your code against a set of rules and flags potential problems, such as syntax errors, undefined variables, and unused code.
- Sentry: Sentry is an error tracking tool that helps you identify and fix issues in your production applications. It provides real-time notifications when errors occur, along with detailed information on the cause of the error and how to fix it.