How to create a Whatsapp Bot with Node + Twilio
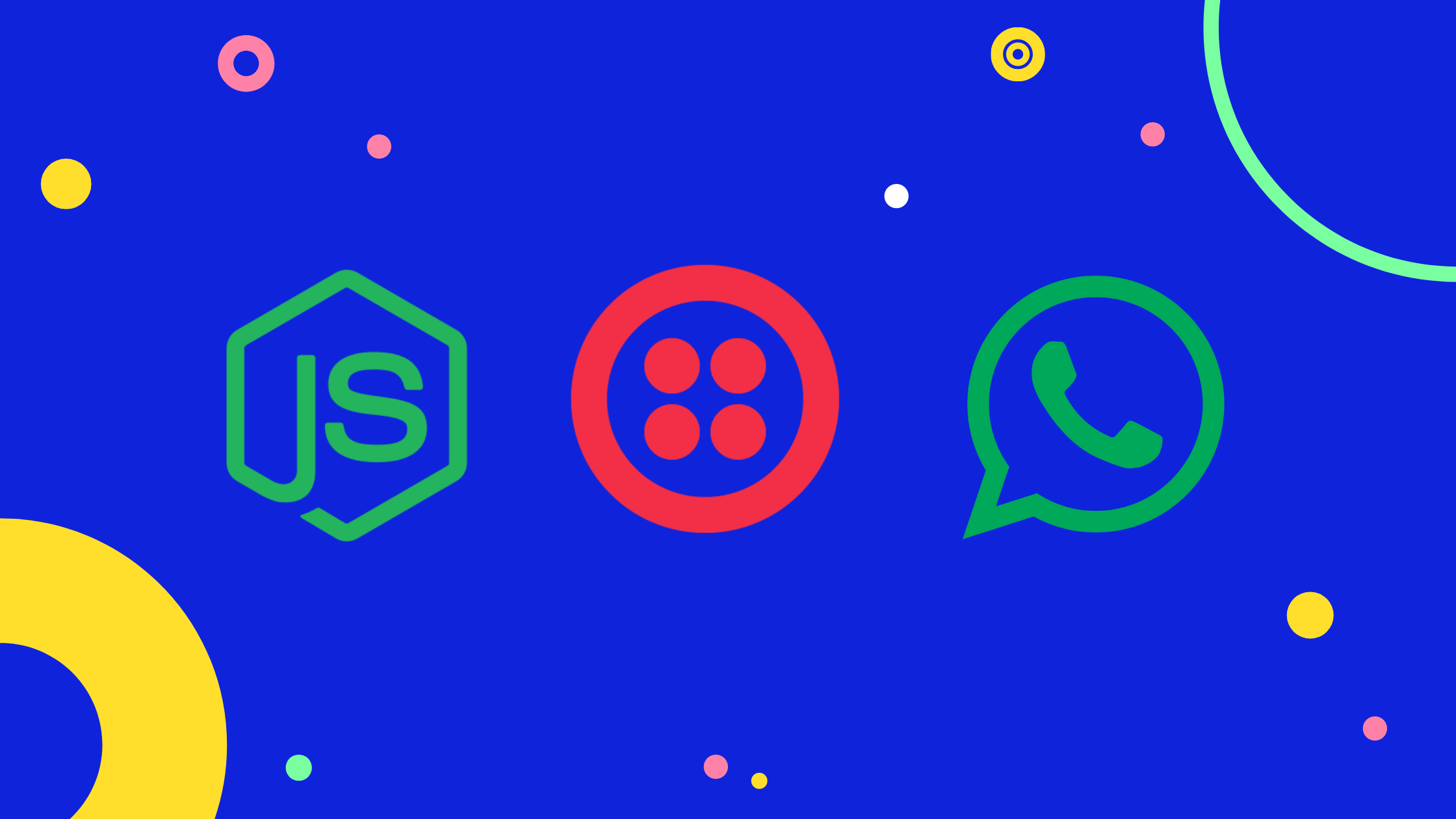
With over 1.5 million users in 180 countries, Whatsapp has grown to be more than just a messaging app, but to be a channel where businesses can easily reach out to their customers. Integrating bots onto the Whatsapp platform offers a golden opportunity for your business to offer conversational commerce and customer support.
To all those a bit lost here, a bot is just software developed to automate tasks you would normally do on your own like fetching and displaying information, making reservations, notifying people of certain events. Now Bots are not a new invention, they have been around for a long time(about 50 years now) but they have only become famous now due to advancements in A.I, a rise in Restful APIs and their integration onto the different social media platforms like Facebook, Twitter and Whatsapp.
Why Create Your Own WhatsApp Bot?

Unlike trying to convince a user to download yet another app on their phone to use your service, Whatsapp has the advantage of being installed on a lot of users' phones (over 2 billion users 🤯). Business can leverage on this and deliver their service via Whatsapp reducing the friction to reach the customer. Another advantage of the Whatsapp platform is that most mobile network operators ( remember most internet users are now mobile) offer special Whatsapp bundles, which users will likely purchase as opposed to data bundles. This means your customers might not afford data to go on the internet, which is a common case for Africa, but they will have data to go onto Whatsapp.
Also, the simplicity of Whatsapp, it's mainly text-based interface, provides a new unique user experience for the user which is likely to be distraction-free. Additionally targeting your customers on the platforms they use daily is more personalized and increases engagement.
NOW TO THE FUN STUFF !!!
A wise man once said talk is cheap, show me the code. In this tutorial, I'll be teaching you how to create a simple bot which replies any message you send to it with a Chuck Norris joke. This is the equivalent of a hello world app, not very useful but a great way to explain the basics.
To follow along, you need to have a Twilio Account in order to access the Whatsapp API, you can create one here. Unfortunately Whatsapp currently doesn't allow direct integration to their API and you have to integrate via one of their "business solution providers". Twilio is the best I've experienced so far. They give you a friendly API and a free sandbox number to test out your bot before going live.  After signing up for Twilio simply go to the Dashboard and navigate  Dashboard>All Products> Programmable SMS> Try Out> Try Out Whatsapp or follow this handy link. After this, you will be taken through an onboarding process for your whats-app sandbox account.
At this stage, we can now begin our coding journey. Head over to the terminal in our project folder and run the following commands
npm init
This creates our package.json file which npm needs to run the NodeJS project.
npm install express axios twilio --save
Here we install dependencies required for the project. We will use Express as our web server, Axios to make requests to our jokes API and Twilio to send and receive Whatsapp messages.
With this out of the way we can go ahead and create our index.js
file which will be the entrypoint of our app and for simplicity its also the only file in our app.
const express = require("express");
const axios = require("axios");
const MessagingResponse = require("twilio").twiml.MessagingResponse;
const port = process.env.PORT || 3000;
const app = express();
app.post("/", (req, res) => {
var twiml = new MessagingResponse();
axios
.get("https://api.chucknorris.io/jokes/random")
.then(function (response) {
console.log(response.data);
twiml.message(response.data.value);
})
.catch(function (error) {
console.log(error);
twiml.message("An error occured, please try again later");
})
.finally(function () {
res.writeHead(200, { "Content-Type": "text/xml" });
res.end(twiml.toString());
});
});
app.listen(port, async () => {
await console.log(`Application successfully running on port: ${port}`);
});
And that's all the code we need. Yes, we have just integrated to Whatsapp with less than 30 lines of code. A bit confused, let me break it down for you.
The first 5 lines define variables we going to use for our small little app. First, we import the required libraries, define our port as either the environment port or 3000 if we are running on localhost, and then define a variable app which is our express server. Normally we have to use router and controller with our express server to handle different routes, but since we only have 1 route and the program is relatively small, I will define it all in the index.js file.
To define our route we call the app.post() method passing in the route "/" which is the default route for the server, i.e localhost:3000/
. The method also takes in a function as its second parameter, this function handles what to do when a request is encountered at this route. The function has 2 parameters a request and a response object i.e (req, res)
, the request object contains data passed to our post endpoint, this will include data such as the message sent to us via Whatsapp and the number of the sender, we however will not be using these in this tutorial as the goal is simply to respond to any message with a Chuck Norris joke. The response object is the one we will use to reply to the message, it exposes methods such as res.writeHead()
to provide header information and res.end()
to provide the body and send back a response.
Inside our handle function the code follows a very simple flow, first we define a twilio style Messaging Response Object
var twiml = new MessagingResponse();
After that we use the powerful Axios library to retrieve a joke from the API. axios.get("https://api.chucknorris.io/jokes/random")
Since Axios is promised based we simply call .then
to get the response. After that we simply make that value our Twilio message
.then(function (response) {console.log(response.data);twiml.message(response.data.value);})
In the case of an error, we simply catch the error and respond with a friendly error message
.catch(function (error) {console.log(error);twiml.message("An error occured, please try again later");})
And then after we have our reply message we simply add our response headers and pass the message back to Twilio as our response in the finally block.
.finally(function () {res.writeHead(200, { "Content-Type": "text/xml" });res.end(twiml.toString());})
And that's all for our handling function. Final piece of the puzzle is to actually start our express server and we do that by running the following 3 lines.
app.listen(port, async () => {
await console.log(`Application successfully running on port: ${port}`);
});
To actually test this our with Twilio we will need a publicly accessible url, we can either get this by deploying to a PAAS like Heroku or run the program locally and use ngrok to tunnel it to the internet over https like a boss😎.
When you get the publicly accessible URL, we can add it on the Twilio dashboard here and replace the provided webhook url for when a message comes in and hit save. After that's done, you can message the Twilio sandbox WA number and receive awesome Chuck Norris jokes courtesy of chucknorris.io.

If you enjoyed this tutorial, please subscribe as this is only an introduction on how to build Whatsapp Bots with Twilio and the next tutorials in the series will be more practical and hands-on.
