Understanding Vector Embeddings: Techniques, Use Cases, and OpenAI's Ada Model
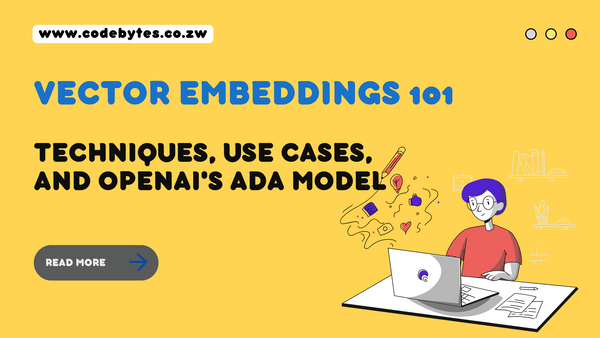
In today's world of information overload, finding relevant and meaningful content quickly is more important than ever. Enter vector embeddings, the unsung heroes of semantic search and modern-day information retrieval. Vector embeddings have numerous applications in software development, such as sentiment analysis, machine translation, semantic search, document clustering, and recommendation systems. But, when you're just starting to explore this fascinating domain, it might seem complicated and a bit daunting.
Fear not! In this article, I will introduce vector embeddings in a simple and engaging way. We'll dive into how embeddings capture the meaning behind words and explore the different techniques used to create them. We'll also learn about how OpenAI's Ada model can be leveraged for generating text embeddings. So, grab a cup of coffee and join in on this exciting journey!
What are Vector Embeddings?
Vector embeddings are numerical representations of words, phrases, or other linguistic elements in a continuous vector space. They capture the semantic relationships between words, allowing algorithms to understand and process natural language more effectively. By representing words as vectors, we can perform mathematical operations on them, making it easier for algorithms to sift through massive amounts of text data and identify meaningful patterns.

Vector embeddings have become a cornerstone in many NLP tasks and applications. By transforming text data into numerical data, developers can create powerful software solutions that understand and interpret human language. Some common applications of vector embeddings in software development include:
- Semantic search engines and chatbots that understand the meaning behind user queries
- Document clustering and categorization for easy navigation
- Automatic tagging and indexing of content
- Personalized content recommendations based on user preferences
- Identifying relationships and patterns in large text corpora
Understanding How Vector Embeddings Work
To truly appreciate the magic of vector embeddings, let's take a closer look at how they work. At a high level, vector embeddings map words to high-dimensional vectors in a way that captures their semantic meaning. Each word is assigned a unique vector, and words with similar meanings have vectors that are close to each other in the vector space. For example, the words "dog" and "puppy" would have similar vectors, while the words "dog" and "banana" would have dissimilar vectors.
The primary goal of vector embeddings is to capture the semantic relationships between words or phrases. In the vector space, these relationships can be represented as geometric properties. For instance, words that are semantically related have vectors that are closer together, while unrelated words have vectors that are further apart. For example, in the vector space, citrus fruits such as oranges, grapes, and lemons are closely related. Non-citrus fruits such as apples are closer in the vector spaces to oranges than non-fruit objects such as pets, billings, and government.

Vector Embeddings and Semantic Search
Semantic search is an advanced approach that aims to understand the intent and contextual meaning of search queries instead of relying solely on keywords. By leveraging vector embeddings and other NLP techniques, semantic search engines can provide more relevant, accurate, and personalized results to users.
Key benefits of semantic search include:
- Improved relevance: Understanding context for better alignment with user intent.
- Natural language understanding: Interpreting complex queries for a more intuitive search experience.
- Personalization: Tailoring search results based on user preferences and contextual factors.
- Disambiguation: Differentiating between homonyms for more accurate results.
Semantic search is especially valuable for information retrieval in large datasets and document stores, where traditional keyword-based methods can struggle to find meaningful relationships and patterns. By comprehending the underlying meaning and relationships between words, semantic search engines can surface relevant content faster and more accurately, even in vast repositories of information.
In addition to enhancing traditional search interfaces, semantic search can be combined with Language Models like ChatGPT to create conversational interfaces that answer user questions in natural language. By leveraging the context provided by semantic search, these chat interfaces can offer more accurate and context-aware responses, resulting in a seamless and engaging user experience. I recently built a project using this very concept for a Civic tech application that can search and answer questions about my Country's constitution. You can check out the project here-> civicguru.co.zw.
Techniques for Creating Vector Embeddings
There are several techniques available for creating vector embeddings. Some of the most popular methods include Word2Vec, GloVe, FastText, and Transformer-based models like BERT and GPT.
a. Word2Vec
Word2Vec is a widely-used technique for generating word embeddings. It comprises two models: Continuous Bag of Words (CBOW) and Skip-Gram. The CBOW model predicts a target word based on its surrounding context words, while the Skip-Gram model predicts context words given a target word. Both models are trained on large text corpora and result in dense vector representations of words.
b. GloVe
GloVe (Global Vectors for Word Representation) is another popular method for generating word embeddings. It combines the benefits of two approaches: matrix factorization and local context window methods. GloVe creates a word co-occurrence matrix from a large text corpus and uses it to learn vector representations that capture the linear relationships between words. The primary goal of GloVe is to generate embeddings that perform well on word analogy tasks.
c. FastText
FastText, developed by Facebook, is an extension of the Word2Vec model that takes into account subword information. Instead of treating each word as an atomic unit, FastText breaks words into character n-grams, allowing the model to learn more fine-grained information about word morphology. This approach is particularly useful for handling out-of-vocabulary words and morphologically rich languages.
d. BERT, GPT, and other Transformer-based models
More recently, Transformer-based models like BERT (Bidirectional Encoder Representations from Transformers) and GPT (Generative Pre-trained Transformer) have gained popularity for generating contextualized word embeddings. Unlike traditional word embeddings, which assign a single vector to each word, these models produce embeddings that are context-dependent, resulting in richer and more dynamic representations of word meanings.
e. Comparing and choosing the right technique
Each of the techniques mentioned above has its strengths and weaknesses, and the choice of method depends on the specific problem at hand. Traditional methods like Word2Vec and GloVe are faster to train and use, making them suitable for smaller datasets and lower-resource settings. On the other hand, Transformer-based models like BERT and GPT offer more powerful and context-aware embeddings, which can lead to better performance in downstream NLP tasks, albeit at the cost of increased computational requirements.
OpenAI's Ada Model for Generating Text Embeddings
OpenAI's Ada model is a variant of the GPT architecture that is designed for a wide range of NLP tasks, including generating text embeddings. Like other GPT models, Ada is pre-trained on a massive corpus of text data and fine-tuned for specific tasks as needed. The Ada model boasts a 10x cost advantage at $0.0004/1k tokens (roughly 3,000 pages per US dollar), making it an attractive option for developers working on semantic search and information retrieval tasks.
Now, let's dive into a step-by-step example of how to use the OpenAI REST API to generate text embeddings with Ada.
- Install the necessary libraries:
pip install requests
2. Import the required modules:
import requests
import json
3. Define your OpenAI key
Obtain an API key from the OpenAI website. Once you have the key you can set it as an environment variable or directly in your code
OPENAI_API_KEY= "your_api_key_here"
4. Create a function to generate embeddings using the text-embedding-ada-002
model and the REST API:
def generate_embeddings(text):
url = "https://api.openai.com/v1/embeddings"
headers = {
"Content-Type": "application/json",
"Authorization": f"Bearer {OPENAI_API_KEY}"
}
data = {
"input": text,
"model": "text-embedding-ada-002"
}
response = requests.post(url, headers=headers, data=json.dumps(data))
return response.json()
5. Call the function with your input text:
text = "Vector embeddings are incredibly useful in natural language processing."
response = generate_embeddings(text)
The response
variable now contains the text embeddings for the input sentence in the following format:
{
"data": [
{
"embedding": [
-0.006929283495992422,
-0.005336422007530928,
...
-4.547132266452536e-05,
-0.024047505110502243
],
"index": 0,
"object": "embedding"
}
],
"model": "text-embedding-ada-002",
"object": "list",
"usage": {
"prompt_tokens": 5,
"total_tokens": 5
}
}
You can access the embeddings by indexing the embedding
key in the data
list, for example: embeddings = response['data'][0]['embedding']
.
Conclusion
In this article, we introduced the concept of vector embeddings, their importance, and applications in the software development field. We also explored how vector embeddings work and provided examples to illustrate the concept. We discussed various techniques for creating vector embeddings, such as Word2Vec, GloVe, FastText, and Transformer-based models like BERT and GPT. We also demonstrated how to generate text embeddings using OpenAI's cost-effective Ada model and the REST API, unlocking a world of possibilities for your NLP projects.
With this newfound knowledge, you're ready to embark on your journey to develop innovative semantic search and information retrieval solutions. Remember to experiment with different techniques and models to find the perfect fit for your specific needs. Happy coding, and may the power of vector embeddings be with you!